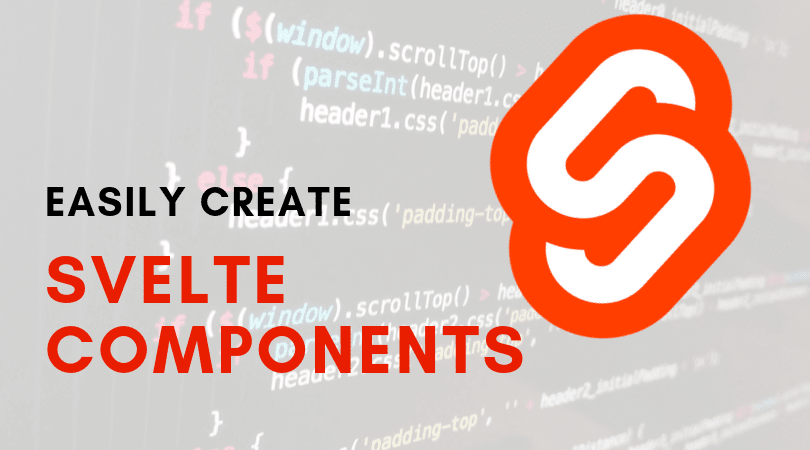
You've probably heard Svelte, a new Javascript "thing" that made buzz and has been a talk of the town in the JS community lately. Early this year, Svelte 3 was out in the public and it gained positive feedbacks from the community. Let's try Svelte and build our reusable components.
What is Svelte?
Svelte is not a UI library nor a complete framework. Svelte, unlike React, Vue, and Angular, is a compiler. It compiles your code directly on build time without needing a virtual DOM; which means that the browser does not need extra work to render your page resulting in fast performance on any applications. Svelte is easy to learn and has good documentation. It gained a lot of praise from the community of developers because of its straightforward and easy to understand docs. Read the introductory blog post here.
Part 1: Let's create a simple reusable button component
To show you how easy to use Svelte, let's make a simple button component.
- Create a Svelte 3 project on CodeSandbox.
Let's use CodeSandbox instead of the typical terminal, for the sake of this tutorial. CodeSandbox is an online IDE with great support for different frameworks like React, Vue, Angular, and of course, Svelte. Go ahead and head over to codesandbox.io and log in using your Github account. Create a sandbox project, choose Svelte on project client templates and create the project.
- Once the project is created, your code looks like this:
- Delete all the code inside Button.svelte. We will start from scratch.
- Let's start by coding a simple button component.
<script></script>
<style></style>
<button>
<slot></slot>
</button>
This is the typical setup for a svelte component or page. The script is added first, followed by the style for styling and lastly, the element(s). See the difference with Vue or React? Svelte doesn't need a parent element or wrappers like a div in React or a template in Vue. This makes the code more readable and straight to the point. We add a slot element inside the button element. Slot contains the value of the child or children of the parent element, which in this case, the text of the button.
- Styling the button component.
Before we start, let's take a moment and think of what properties our button should have. Let's take Twitter Bootstrap as an example of this. Twitter Bootstrap has lots of pre-built design components using classes. For example, the button component has different classes for its color, size, and type. Let's use this idea and add Svelte props to build and design the button component.
The button component has the following attributes:
- Type - a type of button. is it primary? secondary? danger? info?
- Size - the size of the button. Is it small? Medium? Large or extra-large?
- width - width of the button. Is it full width or not?
- Let's make CSS classes for these attributes. Copy and paste this code inside Button. svelte.
<style>
button {
padding: 0.5rem 1rem;
border-radius: 0.25rem;
border: none;
color: white;
}
button:hover {
cursor: pointer;
}
.primary {
background: blue;
}
.secondary {
background: red;
}
.plain {
background: white;
color: black;
}
.outline {
border: 1px solid black;
color: black;
}
.large {
padding: 1rem 2rem;
}
.medium {
padding: .75rem 1.5rem;
}
.full {
width: 100%;
}
.half {
width: 50%;
}
</style>
The code above is quite pretty self-explanatory. They are classes that we need for styling the button component. Here's a brief description of the code above. First, we added padding to the button, gave it a white color, removed the border and added a border-radius and gave a pointer cursor on hover state. Next, we added primary, secondary, plain and outline classes for the type of the button. We also put large and medium classes for the size of the button and lastly the full and half classes for the width of the button.
- Adding Props to our component To make this button reusable, we need to pass values to our component. These values are so-called props or properties. Inside script tag, in Button.svelte file, paste this code:
<script>
export let type = null;
export let size = null;
export let width = null;
</script>
These are properties we need to pass in the component. The default values are null, to avoid errors in logs if we do not use one or more of these properties inside the component. We then need to use these props inside our element as classes.
<button class="{type} {size} {width}">
<slot />
</button>
The complete Button.svelte code looks like this:
<script>
export let type = null;
export let size = null;
export let width = null;
</script>
<style>
button {
padding: 0.5rem 1rem;
border-radius: 0.25rem;
border: none;
color: white;
}
button:hover {
cursor: pointer;
}
.primary {
background: blue;
}
.secondary {
background: red;
}
.plain {
background: white;
color: black;
}
.outline {
border: 1px solid black;
color: black;
}
.large {
padding: 1rem 2rem;
}
.medium {
padding: .75rem 1.5rem;
}
.full {
width: 100%;
}
.half {
width: 50%;
}
</style>
<button class="{type} {size} {width}">
<slot />
</button>
- Let's use the component. Now to use this button component, import this on the App.svelte file and use the properties we added.
<script>
import Button from "./Button.svelte";
</script>
<Button type="primary" size="large" width="full">Primary</Button>
<Button type="secondary" size="medium" width="half">Secondary</Button>
<Button type="plain">Plain</Button>
<Button type="outline">Outline</Button>
You can preview the result inside your Codesandbox project.
Let's make another component.
Part 2: Let's create a reusable Avatar component
- Create Avatar.svelte inside your src project.
- Paste this code inside Avatar.svelte:
<script>
export let type = null;
export let src = null;
export let size = null;
</script>
<style>
img {
width: 3rem;
height: 3rem;
}
.circle {
border-radius: 50%;
}
.square {
border-radius: 5%;
}
.medium {
width: 5rem;
height: 5rem;
}
.large {
width: 8rem;
height: 8rem;
}
</style>
<img {src} alt="{src}" class="{type} {size}">
The avatar component has the following attributes:
- Type - a type of avatar. Is it rounded or not?
- Size - the size of the avatar. Is it Medium? Large?
- Src - The image source
- Let's use the component. Now to use this avatar component, import this on the App.svelte file and use the properties we added.
<script>
import Avatar from "./Avatar.svelte";
...
</script>
<Avatar src="https://github.com/leighayanid.png" />
<Avatar src="https://github.com/leighayanid.png" type="square" size="medium" />
<Avatar src="https://github.com/leighayanid.png" type="circle" size="large" />
You can fork the full code here: https://codesandbox.io/s/lucid-knuth-lhx41?fontsize=14 That is how easy to create components in Svelte. If you want to build more components, the official docs would be of great help to you. I hope you learn something from this tutorial. Till the next blog. Thanks for visiting again.